Mix and match border colors in React Native
Intro
I recently came across a question about how to color one side of a View
’s border a different color than the other three sides.
Fortunately, React Native allows applying different border styles to each of the four sides of a View
.
View
border styles allow styling all four sides together, via something like borderColor: 'teal'
. Specifying a border color for an individual side would then follow the format of border{Side}Color
- e.g. setting the top border color of a View
to 'teal'
would look like this: borderTopColor: 'teal'
.
Here’s a full example setting each border a different color:
<View
style={{
borderWidth: 4,
borderTopColor: 'red',
borderRightColor: 'yellow',
borderBottomColor: 'green',
borderLeftColor: 'blue',
width: 80,
height: 80
}}
/>
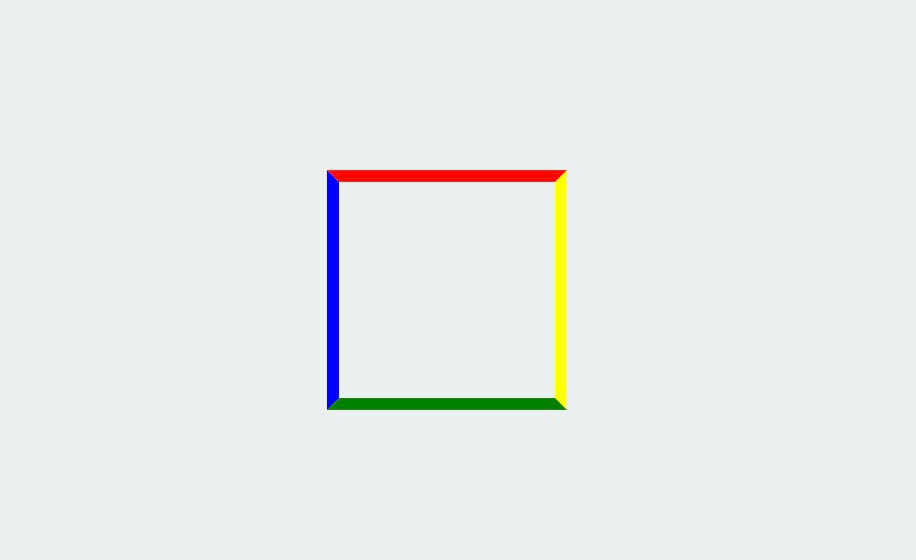
Caveats/Pitfalls
If, unlike the previous example, you want only one side to have a different border color than the others, you may be tempted to combine borderColor
with something like borderRightColor
:
{/* ❌ don't do this */}
<View
style={{
borderWidth: 4,
borderColor: 'green',
borderRightColor: 'yellow', // <-- Won't work on Android, will be green
width: 80,
height: 80,
}}
/>
However this doesn’t seem to work on Android. You’ll have to enumerate the specific color you want for each side of the View
:
{/* ✅ Do this instead */}
<View
style={{
borderWidth: 4,
borderTopColor: 'green',
borderBottomColor: 'green',
borderLeftColor: 'green',
borderRightColor: 'yellow',
width: 80,
height: 80,
}}
/>
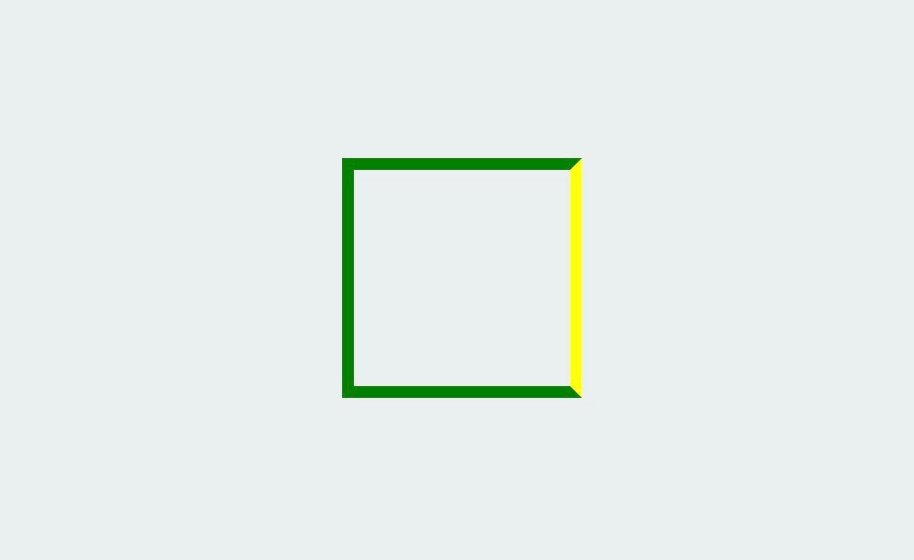
Conclusion
In addition to border colors, React Native View
s support quite a few border styles! For a full list of the different border styles View
supports, check out the official docs for View
’s style props